Convert an image to the closest GBColorPalettes
In this example, the originalImage.jpg has been converted from the png one to jpg format. The conversion has altered the color palette. This example shows how to be sure that the read image has a valid color palette, by setting the convert boolean to True.
Here are the jpg image first, followed by the png one, then by the converted one (visual color difference may be visually difficult to see):
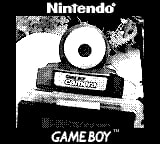
Original Game Boy Camera Image (JPG format)
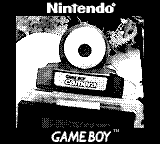
Original Game Boy Camera Image (PNG format)
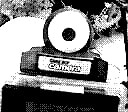
Converted original Game Boy Camera Image (JPG format)
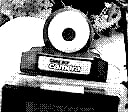
Converted original Game Boy Camera Image (PNG format)
1"""An example to show an image, to log its name and color palette."""
2
3 import cv2
4
5 from gamebeye.gbcamimage.gbcamimage import GBCamImage
6
7 # Path to the image
8 image_filepath = "images\\originalImage.jpg"
9
10 # Creation of an GCCamImage object
11 gb_img = GBCamImage()
12
13 # Reading of the file
14 gb_img.read(image_filepath, convert=True)
15
16 # Logging some info
17 print("Image filename : {}".format(image_filepath))
18 print("GBColorPalette name : {}".format(gb_img.color_palette))
19
20 # Displaying the image
21 title = "GB image with {} color palette".format(gb_img.color_palette.name)
22 cv2.imshow(title, gb_img.data)
23 cv2.waitKey()
24 cv2.destroyWindow(title)